제가 공부한 내용을 정리하는 블로그입니다.
아직 많이 부족하고 배울게 너무나도 많습니다. 틀린내용이 있으면 언제나 가감없이 말씀해주시면 감사하겠습니다😁
스프링 정리를 위한 포스팅입니다.
해당 포스팅은 직렬화, 역직렬화 어노테이션 정리입니다.
직렬화? 역직렬화?
직렬화(Serialization)
직렬화란 객체를 JSON, XML, 바이너리 등 데이터 형식으로 변환하는 과정을 의미합니다.
이 과정을 통해 데이터를 네트워크로 전송하거나 데이터 스트림으로 전송하는데에 사용되빈다.
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws Exception {
User user = new User("testUser", "password123");
ObjectMapper objectMapper = new ObjectMapper();
// 직렬화 (객체 → JSON)
String json = objectMapper.writeValueAsString(user);
System.out.println(json);
}
}
// 출력
{
"username": "testUser",
"password": "password123"
}
역직렬화(Deserialization)
역직렬화란 JSON, XML, 바이너리 데이터를 객체로 변환하는 과정을 의미합니다.
이를 통해 비즈니스 로직에서 객체를 활용하여 사용할 수 있습니다.
import com.fasterxml.jackson.databind.ObjectMapper;
public class Main {
public static void main(String[] args) throws Exception {
String json = "{\"username\":\"testUser\",\"password\":\"password123\"}";
ObjectMapper objectMapper = new ObjectMapper();
// 역직렬화 (JSON → 객체)
User user = objectMapper.readValue(json, User.class);
System.out.println(user.getUsername());
}
}
// 출력
testUser
@JsonIgonre
@JsonIgonre은 JSON 변환 과정에서 특정 필드를 제외할 때 사용됩니다.
import com.fasterxml.jackson.annotation.JsonIgnore;
public class User {
private String username;
@JsonIgnore
private String password; // JSON 변환 시 제외됨
public User(String username, String password) {
this.username = username;
this.password = password;
}
public String getUsername() { return username; }
public String getPassword() { return password; }
}
// 출력
{
"username": "testUser"
}
password 필드가 포함되지 않는 이유가 @JsonIgnore로 때문입니다.
보안 뿐만 아니라 무한 루프 방지 등의 용도로도 사용할 수 있습니다.
@JsonProperty
@JsonProperty는 직렬화 또는 역직렬화할 때 필드의 JSON 키 이름을 변경하는데 사용할 수 있습니다.
특정 조건에서만 JSON 처리를 제어할 수 있기에 더욱 활용도가 높습니다.
import com.fasterxml.jackson.annotation.JsonProperty;
public class User {
private String username;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY) // 역직렬화만 허용
private String password;
}
// 출력
{
"username": "testUser"
}
password는 API 응답에서는 제외되지만, JSON 응답을 받을 때는 사용이 가능합니다.
이렇게 내부적으로 보안을 위한 경우에도 사용할 수 있습니다.
@JsonInclude
@JsonInclude는 특정 조건을 만족하는 필드만 JSON에 포함 할 수 있습니다. 예를 들어 null을 가진 필드를 제외하려면
import com.fasterxml.jackson.annotation.JsonInclude;
@JsonInclude(JsonInclude.Include.NON_NULL)
public class User {
private String username;
private String email;
}
// 출력: email이 null일 때
{
"username": "testUser"
}
와 같이 구현할 수 있습니다.
@JsonIgnoreType
@JsonIgnoreType는 특정 타입의 모든 필드를 JSON 변환에서 제외할 수 있습니다.
객체 내부에 사용되는 숨겨야하는 필드에 대해서 JSON 변환을 제외합니다.
import com.fasterxml.jackson.annotation.JsonIgnoreType;
@JsonIgnoreType
public class SecretData {
public String key = "secret";
}
public class User {
public String username = "testUser";
public SecretData secretData = new SecretData();
}
// 출력
{
"username": "testUser"
}
결과
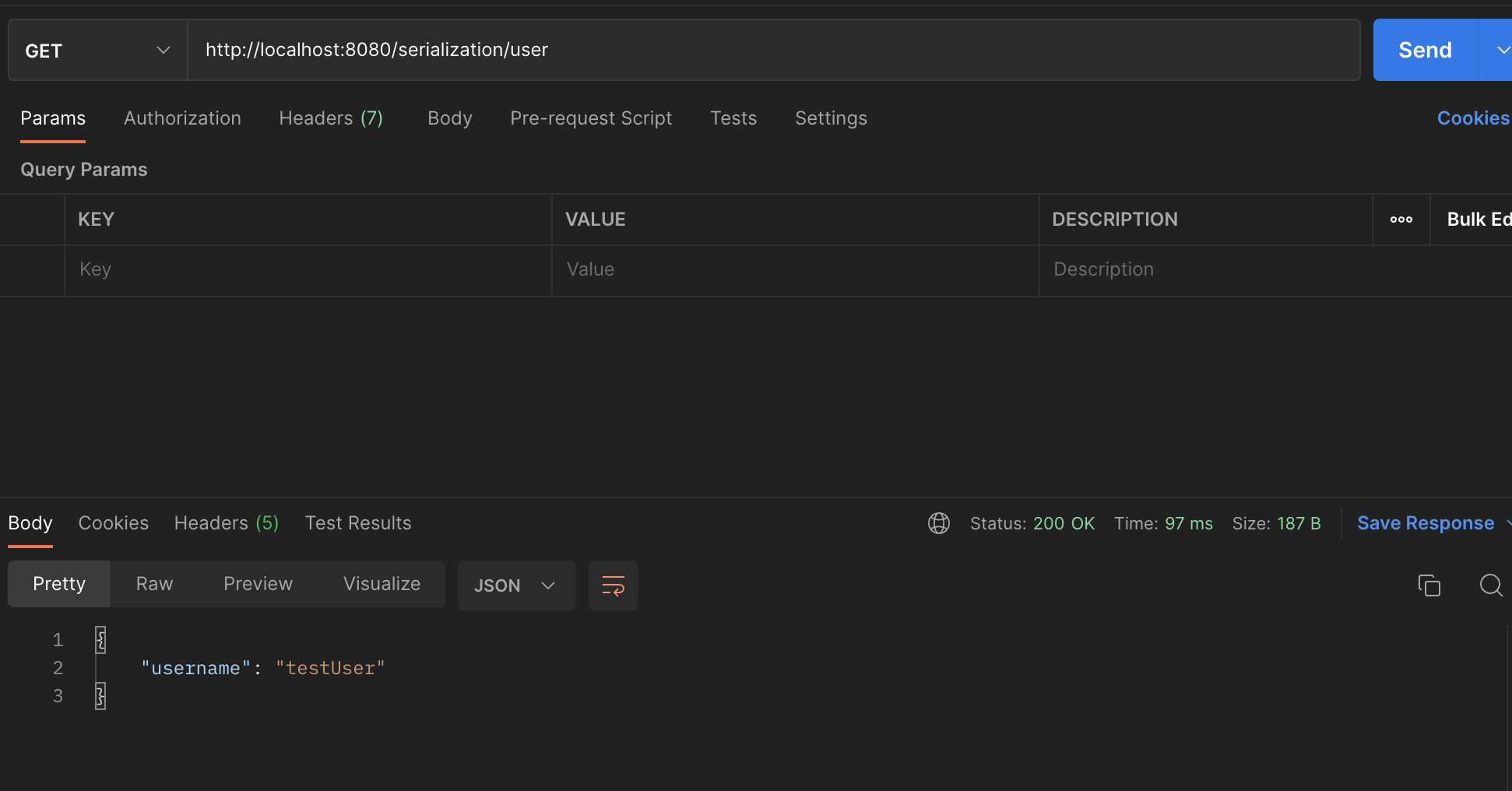
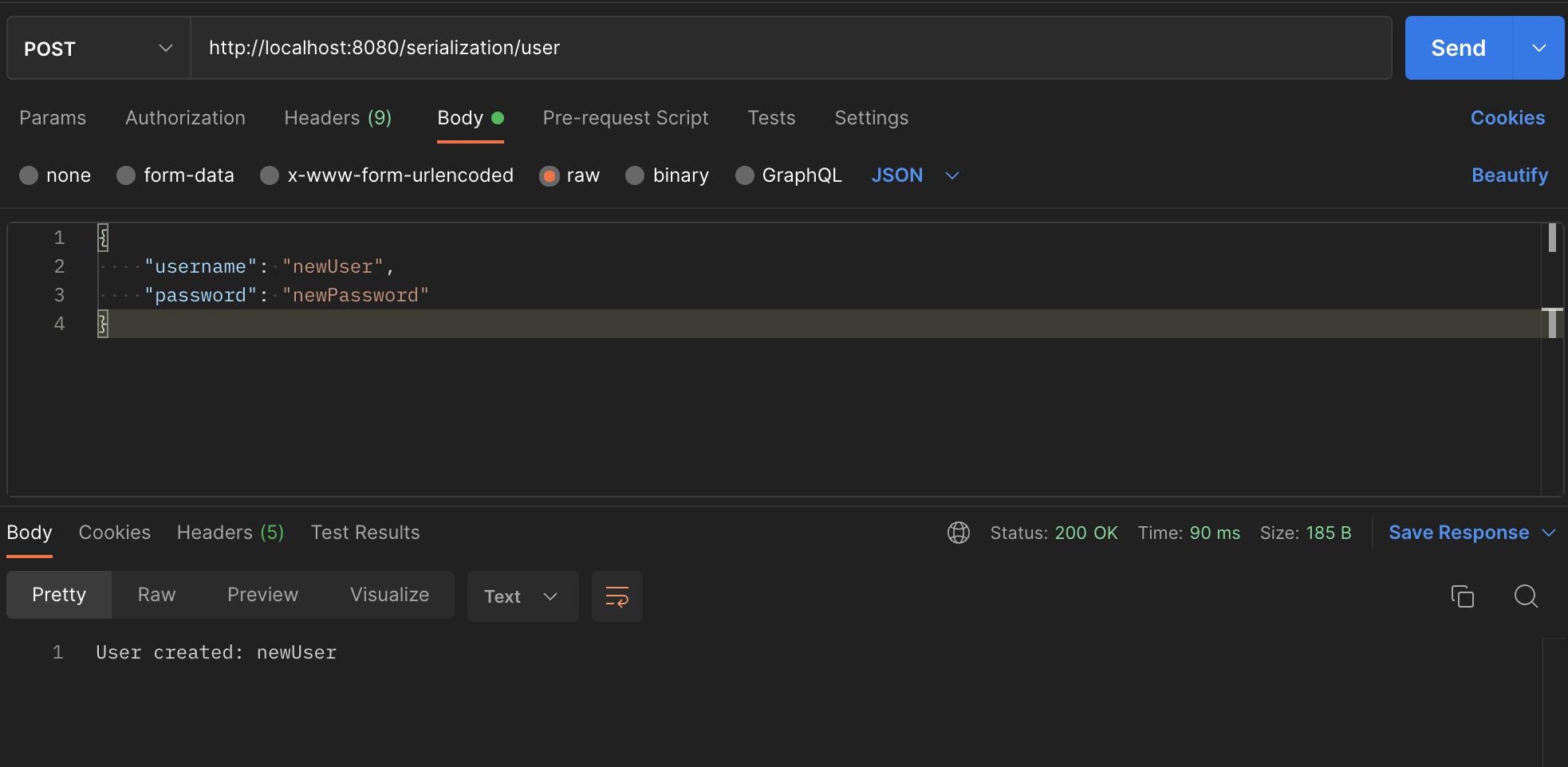
코드
'spring > 어노테이션 정리' 카테고리의 다른 글
[Spring] @Slf4j가 뭐야? (2) | 2025.03.11 |
---|---|
[Spring] @Controller @Service @Repository에 대해 알아보자! (2) | 2024.11.25 |
[Spring] @Lombok에 대해 알아보자! (0) | 2024.11.24 |